Example 2: Merging data from two APIs, and using a private API key
Here we combine data from two free-to-use APIs: Coingecko and CoinMarketCal. CoinMarketCal provides a calendar of upcoming events in the crypto space. However, it requires an api-key to access their data, which can be generated by making an account on their website (https://coinmarketcal.com/).
#pull 10 upcoming events from CoinMarketCal
url = "https://developers.coinmarketcal.com/v1/events"
querystring = {"max":"10"}
payload = ""
headers = {
'x-api-key': coinmarketcal_key,
'Accept-Encoding': "deflate, gzip",
'Accept': "application/json"
}
response = requests.request("GET", url, data=payload, headers=headers, params=querystring)
data = pd.DataFrame(response.json()['body'])
#Paginate through the ten events, and pull data on the first coin mentioned in each event from Coingecko.
#Skip cases where the name returned from coinmarketcal is not an available ID in coingecko.
output = pd.DataFrame(columns = ["coin", "event", "date","coin_description", "mc_change"])
for i in range(len(data)):
try:
coin = data.iloc[i].coins[0]['id']
cg_info = requests.get(f"https://api.coingecko.com/api/v3/coins/{coin}" ).json()
coin_description = cg_info['description']['en']
mc_change = cg_info['market_data']['market_cap_change_percentage_24h']#['usd']
output.loc[len(output) + 1] = [coin, data.iloc[i].title['en'], data.iloc[i]['date_event'],coin_description,mc_change ]
except:
pass
result = output.to_json(orient = 'records')
Here, we get a list of 10 events from CoinMarketCal. Next, we loop through these 10 events, pulling price change and coin descriptions from Coingecko. We store this data in a dataframe called "output".
It is important to note that we store our API key for CoinMarketCal as a private variable. This ensures the security of our key.
Next, we convert our output into a json object called "result" that can be used by the component builder to make a table.
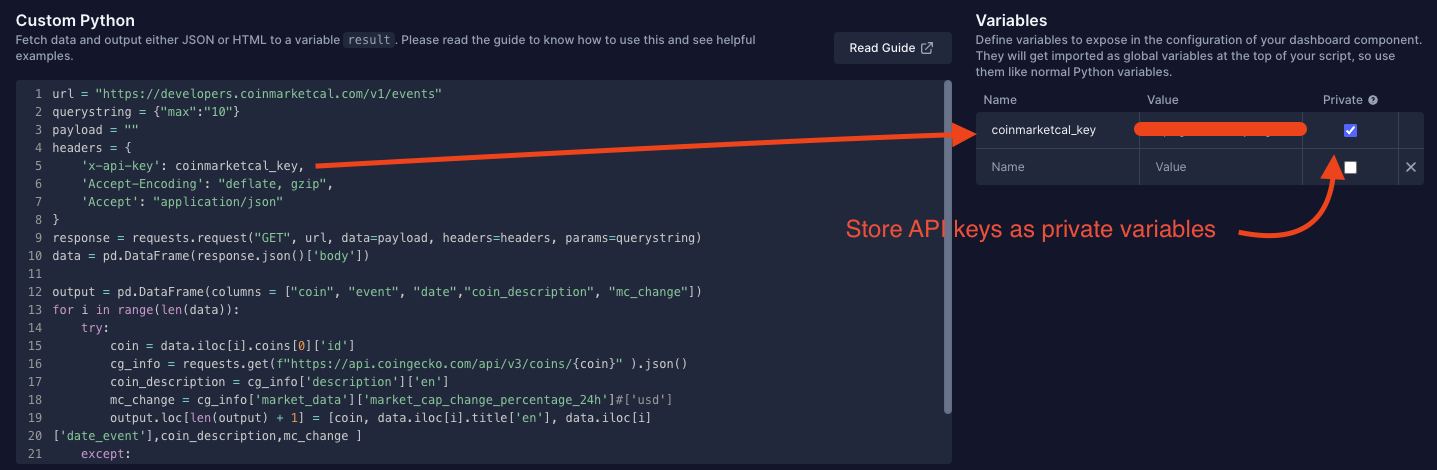
Lastly we configure our parameters for a table by naming each column selected and selecting a format type. Note: we use the Pos/Neg Percent as the format type for price change and date as the type for the event date.
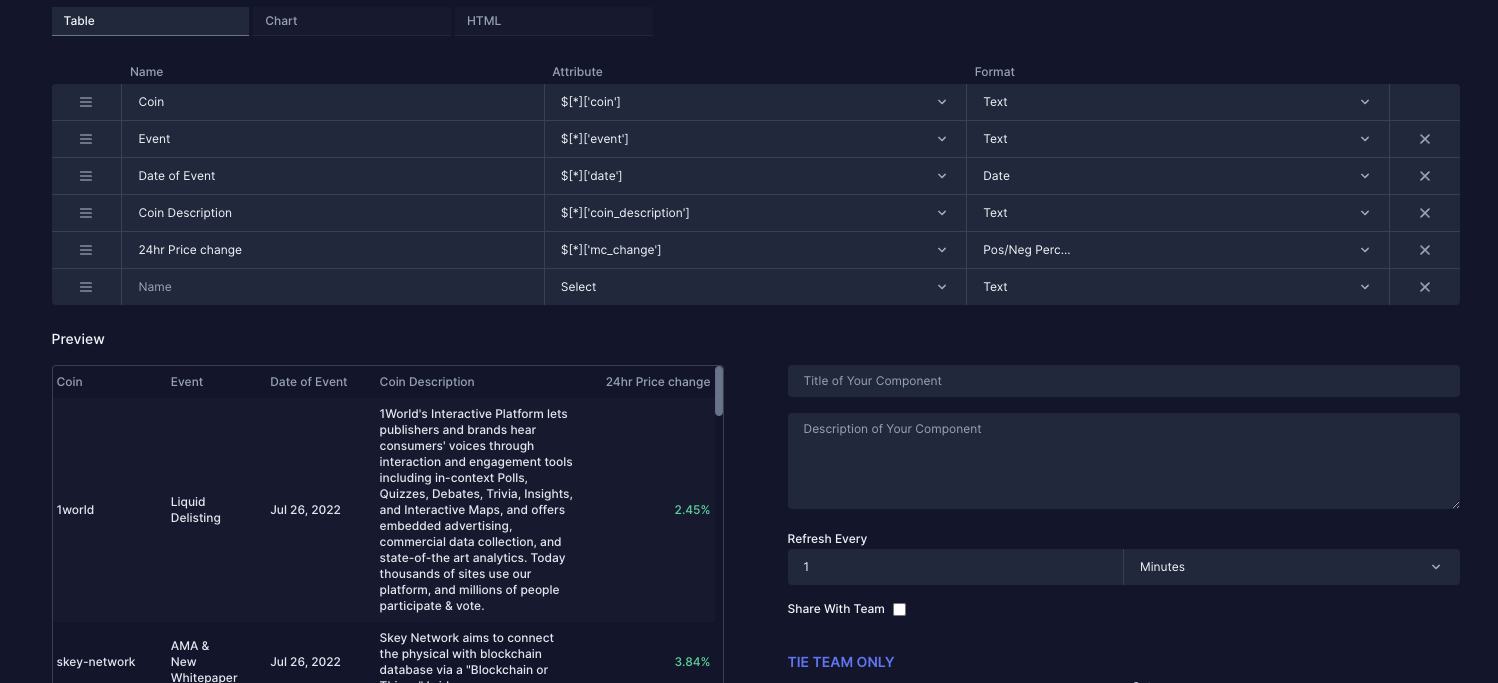
Updated over 2 years ago